Variable is name of memory location, it is an entity that may be change during program execution. There are three types of variables :-
1. Local variable
2. Instance variable
3. Static variable
In order to use a variable in a program you to need to perform 2 steps :-
- Variable Declaration
- Variable Initialization
Examples of other Valid Declarations are :-
int a,b,c;
float pi;
double d;
char a;
To initialize a variable, you must assign it a valid value.
double d0=20.22d;
char 'a';
There are three types of variables :-
1. Local variable
2. Instance variable
3. Static variable
1.Local Variable :- Variable that is declared inside the method is called local variable.
2.Instance Variable :- Variable that is declared inside the class but outside the method is Called as instance variable.
3.Static variable :- Variable that is declared as static is called static variable.
Example :-
class A {
int data = 99; //instance variable
static int a = 1; //static variable
void method() {
int b = 90; //local variable
}
}
Data Types in Java :-
- Primitive Data Types
- Non-primitive Data Types
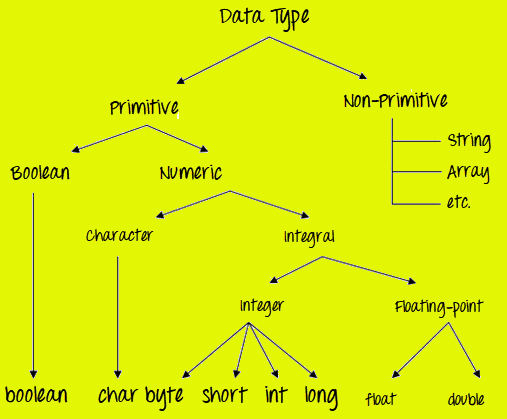
Primitive Data Types
Primitive Data Types are predefined and available within the Java language. Primitive values do not share state with other primitive values.
Data Type | Default Value | Default size |
---|---|---|
byte | 0 | 1 byte |
short | 0 | 2 bytes |
int | 0 | 4 bytes |
long | 0L | 8 bytes |
float | 0.0f | 4 bytes |
double | 0.0d | 8 bytes |
boolean | false | 1 bit |
char | '\u0000' | 2 bytes |
Comments
Post a Comment